Exploring Tuple Addition in Python A Guide to Effortlessly Combine Tuples
3 min read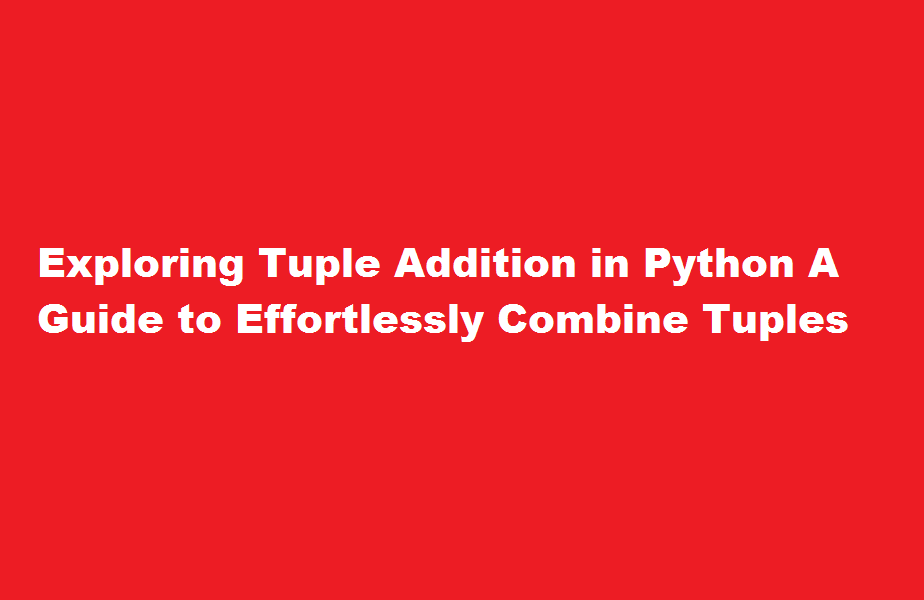
Introduction
Python, being a versatile programming language, offers several built-in data structures to handle complex operations. Tuples, one such immutable data structure, provide a convenient way to store and access multiple elements. While tuples cannot be modified directly, they can be combined to create new tuples. In this article, we will delve into the concept of tuple addition in Python, exploring various methods to merge two tuples and highlighting their appropriate use cases. Whether you’re a beginner or an experienced Python programmer, this guide will help you master the art of tuple addition.
Understanding Tuples
Before we dive into tuple addition, it’s essential to understand the fundamentals of tuples. A tuple is an ordered collection of elements, enclosed in parentheses. Tuples can store different types of data, including numbers, strings, or even other tuples. The key characteristic of tuples is their immutability, meaning once created, their values cannot be changed. This immutability guarantees data integrity and makes tuples suitable for scenarios where data should remain constant throughout the program execution.
Tuple Addition Methods
In Python, there are multiple ways to add two tuples together. Let’s explore some of the most common methods:
Using the ‘+’ Operator
The simplest way to combine two tuples is by using the ‘+’ operator. By concatenating two tuples with ‘+’, a new tuple is created, containing all the elements from both input tuples.
Example
“`
tuple1 = (1, 2, 3)
tuple2 = (‘a’, ‘b’, ‘c’)
new_tuple = tuple1 + tuple2
print(new_tuple)
“`
Output:
“`
(1, 2, 3, ‘a’, ‘b’, ‘c’)
“`
Using the ‘tuple()’ Function
Another approach is to convert both tuples into lists, perform list addition, and then convert the resulting list back into a tuple using the ‘tuple()’ function.
Example
“`
tuple1 = (1, 2, 3)
tuple2 = (‘a’, ‘b’, ‘c’)
new_tuple = tuple(list(tuple1) + list(tuple2))
print(new_tuple)
“`
Output:
“`
(1, 2, 3, ‘a’, ‘b’, ‘c’)
“`
Using Tuple Unpacking
Tuple unpacking is a concise way to merge two tuples. It involves assigning each element of the input tuples to separate variables and then creating a new tuple with the combined values.
Example
“`
tuple1 = (1, 2, 3)
tuple2 = (‘a’, ‘b’, ‘c’)
new_tuple = (*tuple1, *tuple2)
print(new_tuple)
“`
Output:
“`
(1, 2, 3, ‘a’, ‘b’, ‘c’)
“`
Choosing the Right Method
When selecting a method for tuple addition, consider the requirements of your specific use case. If simplicity is a priority, using the ‘+’ operator is straightforward and effective. However, if you need to perform additional operations on the elements, converting the tuples to lists might offer more flexibility. Tuple unpacking, on the other hand, provides a concise syntax, making it suitable for concise code or assignments.
Frequently Asked Questions
What is the function to add two tuples?
When it is required to add the tuples, the ‘amp’ and lambda functions can be used. The map function applies a given function/operation to every item in an iterable (such as list, tuple). It returns a list as the result.
How do you add two tuples in Python?
Operators can be used to concatenate or multiply tuples. Concatenation is done with the + operator, and multiplication is done with the * operator. Because the + operator can concatenate, it can be used to combine tuples to form a new tuple, though it cannot modify an existing tuple.
Conclusion
By exploring the different methods of tuple addition in Python, you can now confidently merge two tuples and create new ones. Whether you opt for the ‘+’ operator, the ‘tuple()’ function, or tuple unpacking, each method has its advantages depending on the specific use case. Understanding these techniques empowers you to work with tuples effectively and efficiently in your Python programs. Experiment with these methods and combine them with other tuple operations to unlock the full potential of tuples as a powerful data structure in Python.
Read Also : Mastering Tuple Input in Python A Comprehensive Guide