Creating Arrays of Objects in Java A Comprehensive Guide
4 min read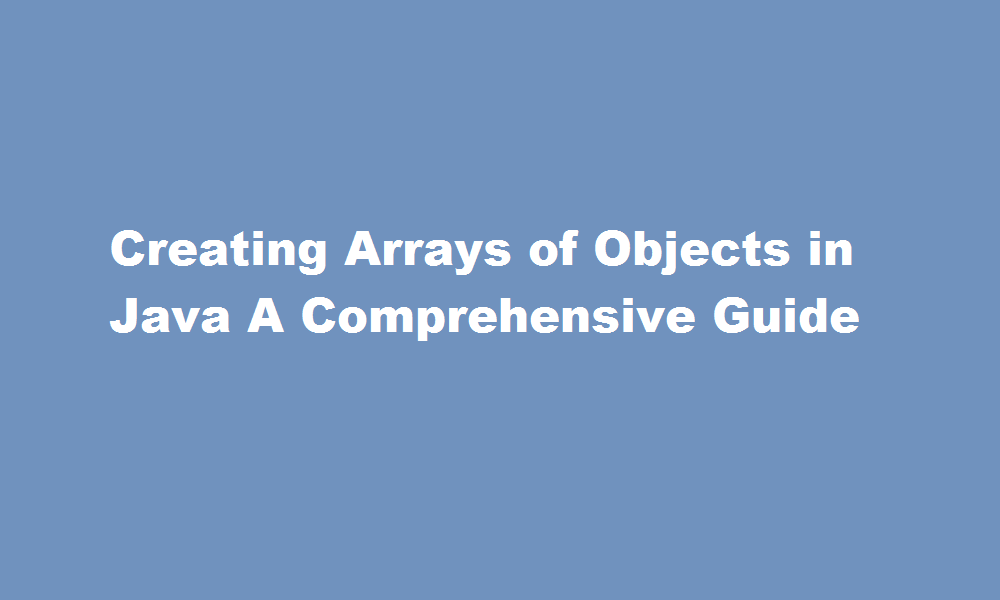
Introduction
In Java, arrays are widely used data structures that allow you to store multiple values of the same type. While arrays are commonly used to store primitive data types, they can also hold objects. This article aims to provide a comprehensive guide on creating arrays of objects in Java. By understanding the fundamentals and following best practices, you will be equipped to leverage the power of arrays to efficiently manage collections of objects in your Java programs.
Object-Oriented Basics
Before diving into arrays of objects, let’s review the fundamental concepts of object-oriented programming (OOP). In Java, objects are instances of classes, which serve as blueprints for creating objects. Each object has its own set of properties (attributes) and behaviors (methods). To create an array of objects, you need to define a class that represents the type of objects you want to store. The class should encapsulate the necessary data fields and provide appropriate methods to manipulate and access the objects’ state.
Declaring an Array of Objects
To declare an array of objects, you first need to declare an array variable, just like you would for arrays of primitive types. The variable holds a reference to the array object. For example, to declare an array of Person objects, you would write
java
Copy code
Person[] people;
This statement declares an array variable named people that can hold references to objects of the Person class. However, at this point, the array is still uninitialized and doesn’t have any objects associated with it.
Creating Objects and Initializing the Array
To create objects and initialize the array, you need to allocate memory and assign the objects to the array elements. Each element of the array is a reference to an object. You can create objects using the new keyword and assign them to the array elements individually or in a loop. Here’s an example that creates three Person objects and assigns them to the array
java
Copy code
people = new Person[3];
people[0] = new Person(“Alice”);
people[1] = new Person(“Bob”);
people[2] = new Person(“Charlie”);
In this example, we first allocate memory for three Person objects. Then, we create individual Person objects using the new keyword and assign them to the respective array elements.
Accessing and Manipulating Objects in the Array
Once you have created an array of objects, you can access and manipulate the objects using the array index. For example, to access the name attribute of the second Person object in the array, you would write
java
Copy code
String secondPersonName = people[1].getName();
In this example, people[1] retrieves the reference to the second Person object, and getName() accesses its name attribute. Similarly, you can call other methods or modify object attributes as required.
Best Practices and Considerations
When working with arrays of objects in Java, it’s essential to keep a few best practices in mind. First, ensure that you initialize the array before accessing its elements; otherwise, you’ll encounter a NullPointerException. Secondly, consider using loops for array initialization and manipulation to avoid repetitive code. Additionally, remember that arrays have a fixed size once initialized, so you cannot dynamically add or remove objects. If you need dynamic resizing, consider using other data structures like ArrayList.
FREQUENTLY ASKED QUESTIONS
What is an array of objects?
An array of objects, all of whose elements are of the same class, can be declared just as an array of any built-in type. Each element of the array is an object of that class. Being able to declare arrays of objects in this way underscores the fact that a class is similar to a type.
What is the syntax for an array of objects?
We can use any of the following statements to create an array of objects. Syntax: ClassName obj[]=new ClassName[array_length]; //declare and instantiate an array of objects.
Conclusion
Arrays of objects in Java provide a powerful way to manage collections of related objects. By understanding the basics of object-oriented programming and following the steps outlined in this article, you can create and manipulate arrays of objects efficiently. Remember to initialize the array, create objects, and access their properties using array indices. Be mindful of best practices, such as using loops for repetitive tasks and considering alternative data structures for dynamic resizing. Armed with this knowledge, you can confidently leverage arrays of objects to enhance the functionality and organization of your Java programs.
Read Also : Mastering Excel The Ultimate Guide to Duplicating Sheets