Harnessing the Power of Vectors in C++ A Comprehensive Guide
3 min read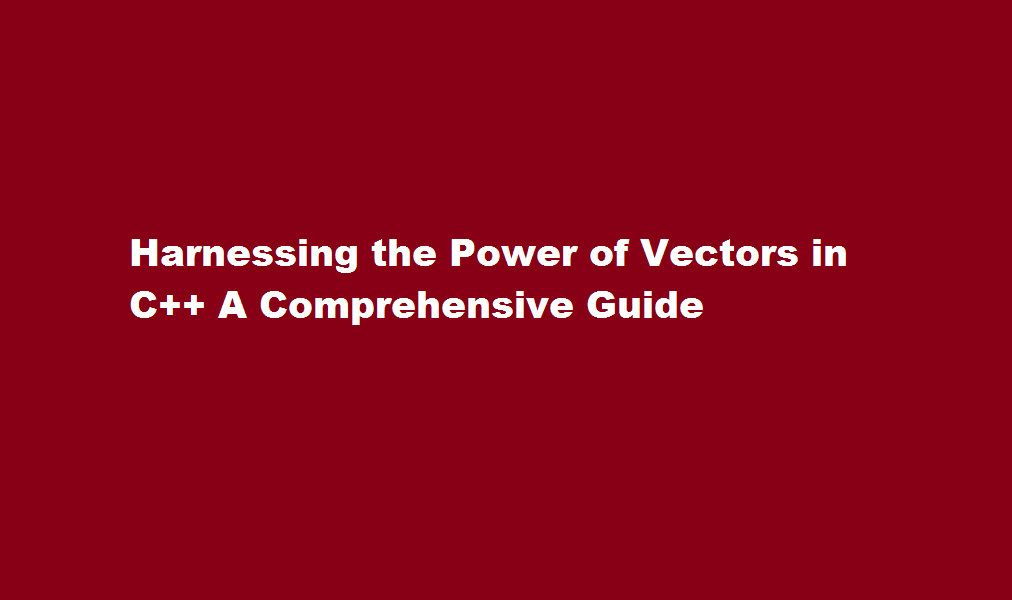
Introduction
C++ is a versatile programming language widely used for its ability to handle complex data structures. One such powerful data structure is the vector, which provides a dynamic array-like functionality while offering additional features. In this article, we will explore the various aspects of vectors in C++, starting from their definition and initialization to performing common operations like insertion, deletion, and searching. By the end, you will have a solid understanding of how to utilize vectors effectively in your C++ programs, enabling you to write efficient and flexible code.
Understanding Vectors
In C++, a vector is a sequence container that can dynamically resize itself to accommodate varying numbers of elements. It provides similar functionality to arrays but offers significant advantages such as automatic memory management and built-in methods for easy manipulation. To include vectors in your C++ program, you need to include the <vector> header file, which contains the necessary vector-related definitions and functions. Once included, you can start working with vectors and take full advantage of their versatility.
Declaring and Initializing Vectors
To declare a vector, you specify its data type, followed by the name you choose. For example, to create a vector of integers, you would write:
cpp
Copy code
std::vector<int> myVector;
You can also initialize vectors during declaration by specifying the elements inside curly braces. For instance:
cpp
Copy code
std::vector<int> myVector = {1, 2, 3, 4, 5};
Additionally, you can use the vector constructor to define a vector with a specific number of elements or initialize it with repeated values.
Insertion and Deletion
Vectors provide efficient ways to insert and delete elements at various positions. To add an element at the end of a vector, you can use the push_back() function, which automatically resizes the vector if necessary. Alternatively, you can insert elements at specific positions using the insert() function. To remove elements, you can use the pop_back() function to delete the last element, or the erase() function to remove an element at a particular index or a range of elements.
Accessing and Modifying Elements
You can access vector elements using the indexing operator ([]), providing the desired index. Vectors also provide methods like at() and front() to access the element at a specific index or the first element, respectively. To modify elements, you can assign new values using the assignment operator (=) or utilize the vector methods, such as assign() or emplace(), to replace or insert elements at desired positions.
Searching and Sorting
Vectors support efficient searching and sorting operations. You can use the find() function to search for a specific value within the vector and retrieve its index. Sorting a vector can be accomplished using the sort() function from the <algorithm> header, which arranges the elements in ascending order by default. For custom sorting requirements, you can provide a comparison function. Other operations like reverse sorting, finding the minimum or maximum element, or finding the occurrence count of an element are also readily available.
FREQUENTLY ASKED QUESTIONS
Do you need to include vectors C++?
vector (or, by its full name, std::vector ) is itself implemented in C++. By writing #include <vector> , you are telling the compiler to not only use your own code, but to also compile a file called vector .
What is vector size () in C++?
The C++ function std::vector::size() returns the number of elements present in the vector.
Conclusion
Incorporating vectors in your C++ programs empowers you with a flexible and efficient data structure that simplifies dynamic array management. By grasping the concepts and techniques discussed in this article, you can harness the power of vectors to optimize your code and build robust applications in C++
Read Also : A Step-By-Step Guide to Including Drop-Down Lists in Excel