Demystifying Date Parsing in Java A Comprehensive Guide
4 min read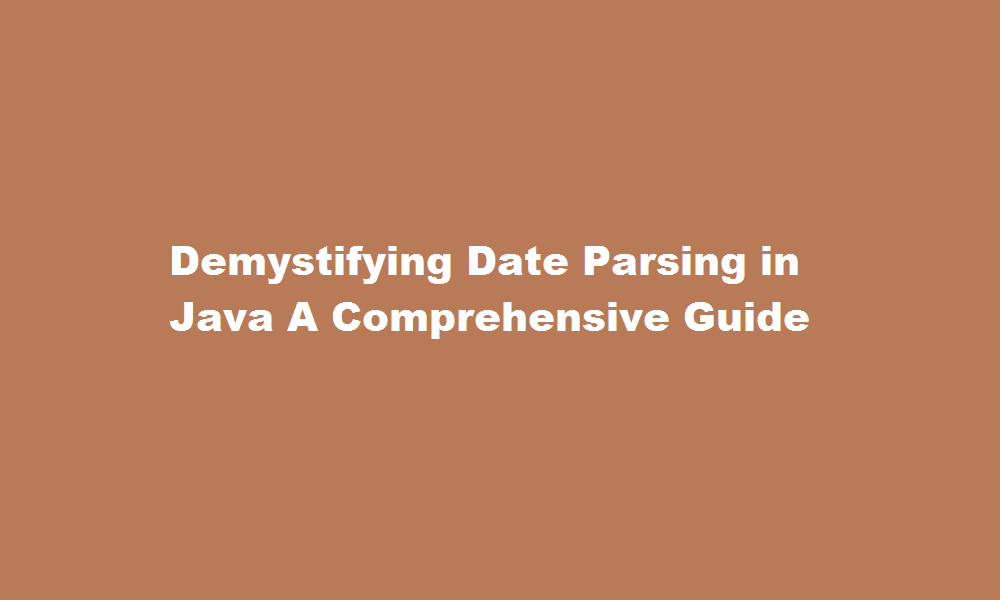
Introduction
Date parsing is a fundamental operation in Java, allowing developers to convert textual representations of dates into objects that can be manipulated and formatted. While it may seem like a simple task, parsing dates accurately and efficiently requires understanding the intricacies of Java’s date parsing mechanisms. In this article, we will explore the principles of date parsing in Java, delve into the core libraries and classes involved, and provide practical examples to guide you through the process.
Understanding Date Formats
Before diving into date parsing in Java, it is crucial to comprehend the concept of date formats. A date format represents the pattern of characters used to represent a date in a textual form. Common examples include “dd/MM/yyyy” for a date in the format of day/month/year, or “yyyy-MM-dd” for a date in the format of year-month-day. Java follows the pattern-based approach for defining date formats, where each character represents a specific element of a date (e.g., ‘d’ for day, ‘M’ for month, ‘y’ for year).
The SimpleDateFormat Class
In Java, the primary class used for parsing dates is SimpleDateFormat, which belongs to the java.text package. This class provides methods to parse a string representation of a date into a Date object. It utilizes a pattern string that defines the expected format of the input date string. The pattern string consists of a combination of date format symbols and literal characters.
Parsing Dates with SimpleDateFormat
To parse a date using SimpleDateFormat, follow these steps
- Create an instance of SimpleDateFormat, passing the desired pattern string as a constructor argument.
- Invoke the parse() method, providing the input date string to be parsed.
- Handle potential exceptions that may occur, such as ParseException, which indicates an invalid or incompatible date format.
- If the parsing is successful, the method will return a Date object representing the parsed date.
Handling Localization and Time Zones
Java provides support for parsing dates based on different locales and time zones. The SimpleDateFormat class allows you to set the desired locale and time zone to ensure accurate parsing. By default, it uses the system’s default locale and time zone. However, you can explicitly set these properties using the setLocale() and setTimeZone() methods, respectively.
Java 8’s DateTimeFormatter
In Java 8 and later versions, a new date and time API, located in the java.time package, was introduced. This API provides the DateTimeFormatter class, which offers enhanced date parsing capabilities. The DateTimeFormatter class uses a different pattern syntax, called the DateTimeFormatter patterns, to define date formats.
Parsing Dates with DateTimeFormatter
To parse dates using DateTimeFormatter, follow these steps
- Create an instance of DateTimeFormatter, passing the desired pattern string as a method argument.
- Use the parse() method of the DateTimeFormatter class, providing the input date string to be parsed.
- Handle potential exceptions, such as DateTimeParseException, which indicates an invalid or incompatible date format.
- If the parsing is successful, the method will return a LocalDateTime object representing the parsed date and time.
FREQUENTLY ASKED QUESTIONS
What is the parse method in date?
parse() parses a date string and returns the time difference since January 1, 1970. parse() returns the time difference in milliseconds.
What is the difference between format date and parse date?
Notice that formatting means converting date to string and parsing means converting string to date.
What is the difference between yyyy and yyyy in Java date format?
A common mistake is to use YYYY. yyyy specifies the calendar year whereas YYYY specifies the year (of “Week of Year”), used in the ISO year-week calendar. In most cases, yyyy and YYYY yield the same number, however they may be different. Typically you should use the calendar year.
Conclusion
Parsing dates in Java is a vital skill for any developer working with date and time data. In this article, we explored the key concepts of date parsing, focusing on the SimpleDateFormat class for Java versions prior to Java 8 and the DateTimeFormatter class introduced in Java 8. By understanding the intricacies of date formats, utilizing the appropriate classes, and handling localization and time zones, developers can confidently parse dates in Java, ensuring accurate and reliable date manipulation in their applications.
Read Also : A Comprehensive Guide to Partitioning an SSD in Windows 11