A Comprehensive Guide To Creating Arrays in Java
3 min read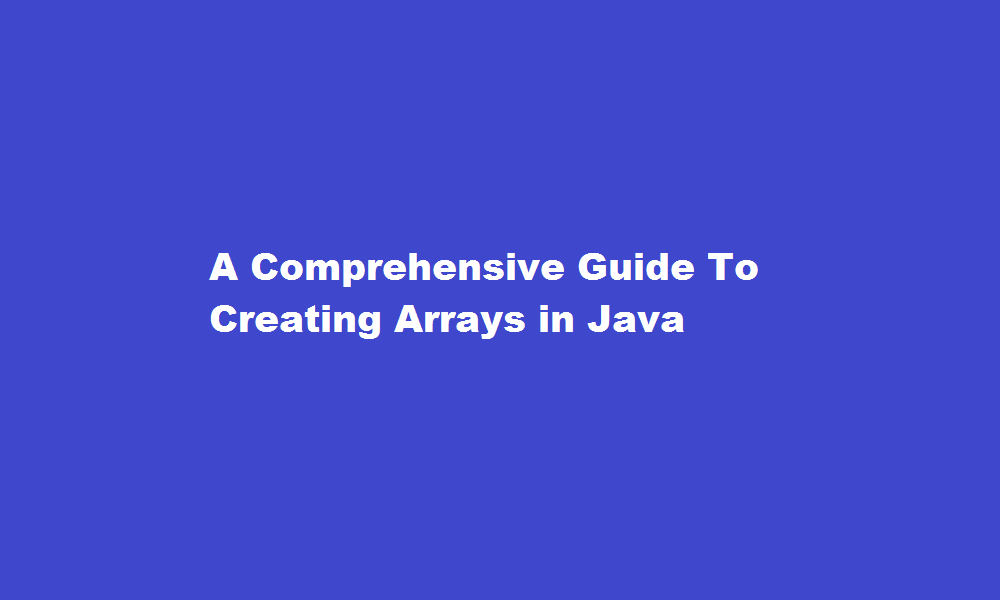
Introduction
Arrays are fundamental data structures in Java that allow you to store and manipulate collections of elements efficiently. Whether you are a beginner or an experienced Java programmer, understanding how to create and utilise arrays is essential. In this article, we will explore the step-by-step process of creating arrays in Java, discuss different types of arrays, and provide practical examples to solidify your understanding.
Declaring an Array
To create an array in Java, you must first declare its type and name. The syntax for declaring an array is as follows
java
Copy code
dataType[] arrayName;
Here, dataType represents the type of elements the array will store, such as int, double, or String. For example, to create an array of integers named numbers, you would use
java
Copy code
int[] numbers;
You can also declare multiple arrays of the same type in a single statement, separating each array name with a comma. It would look like this
java
Copy code
int[] array1, array2, array3;
Remember that at this stage, you have only declared the array; it does not have any memory allocated for elements yet.
Initialising an Array
After declaring an array, you need to initialise it with memory space to store the elements. There are several ways to initialise an array in Java:
Static Initialization In this method, you directly assign values to the array elements when declaring it. For example
java
Copy code
int[] numbers = {1, 2, 3, 4, 5};
Dynamic Initialization With dynamic initialization, you specify the size of the array using the new operator and then assign values to individual elements using an assignment statement. For instance:
java
Copy code
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
Anonymous Array Initialization This approach allows you to declare and initialise an array without assigning it to a variable. It is useful for one-time usage scenarios. For example:
java
Copy code
System.out.println(“Sum: ” + Arrays.stream(new int[]{1, 2, 3}).sum());
Array Length and Indexing
Arrays in Java have a fixed length that is determined during initialization and cannot be changed later. You can access individual elements of an array using zero-based indexing. For example, to access the first element of the numbers array declared earlier, you would use:
java
Copy code
int firstNumber = numbers[0];
It’s important to note that attempting to access an index outside the bounds of the array will result in an ArrayIndexOutOfBoundsException error.
Working with Multidimensional Arrays
Java also supports multidimensional arrays, which are arrays of arrays. You can create two-dimensional, three-dimensional, or even higher-dimensional arrays. For instance, a two-dimensional array of integers named matrix can be created as follows
java
Copy code
int[][] matrix = new int[3][3];
To access or modify elements in a multidimensional array, you need to provide the indices for each dimension. For example, to access the element in the second row and third column of the matrix, you would use
java
Copy code
int element = matrix[1][2];
FREQUENTLY ASKED QUESTIONS
What is an Array?
Array is a collection of similar data types. It can not have different data types. It can hold both primitive types (int, float, double) and object references.
Can you declare an Array without Array size?
No, you can not declare Array without Array size. You will get a compile time error.
Where does Array stored in JVM memory ?
Array is an object in java. So, Array is stored in heap memory in JVM.
Conclusion
Arrays are versatile data structures in Java that provide an organised way to store and manipulate collections of elements. By understanding the process of creating and using arrays, you can write more efficient and structured Java programs.
In this article, we covered the basics of creating arrays in Java, including declaration, initialization, length, indexing, and working with multidimensional arrays. Armed with this knowledge, you can confidently leverage arrays in your Java programming endeavours.
Read Also : A Comprehensive Guide To Creating A Database in MySQL